Widgets
Embed the power of Webex in your web applications ✨
anchorWidgets Overview
anchorWebex Widgets provide Webex native application functionality that can be embedded into any web-based application. They are built with React, Redux, RxJS and the Browser SDK to communicate with the Webex Platform.
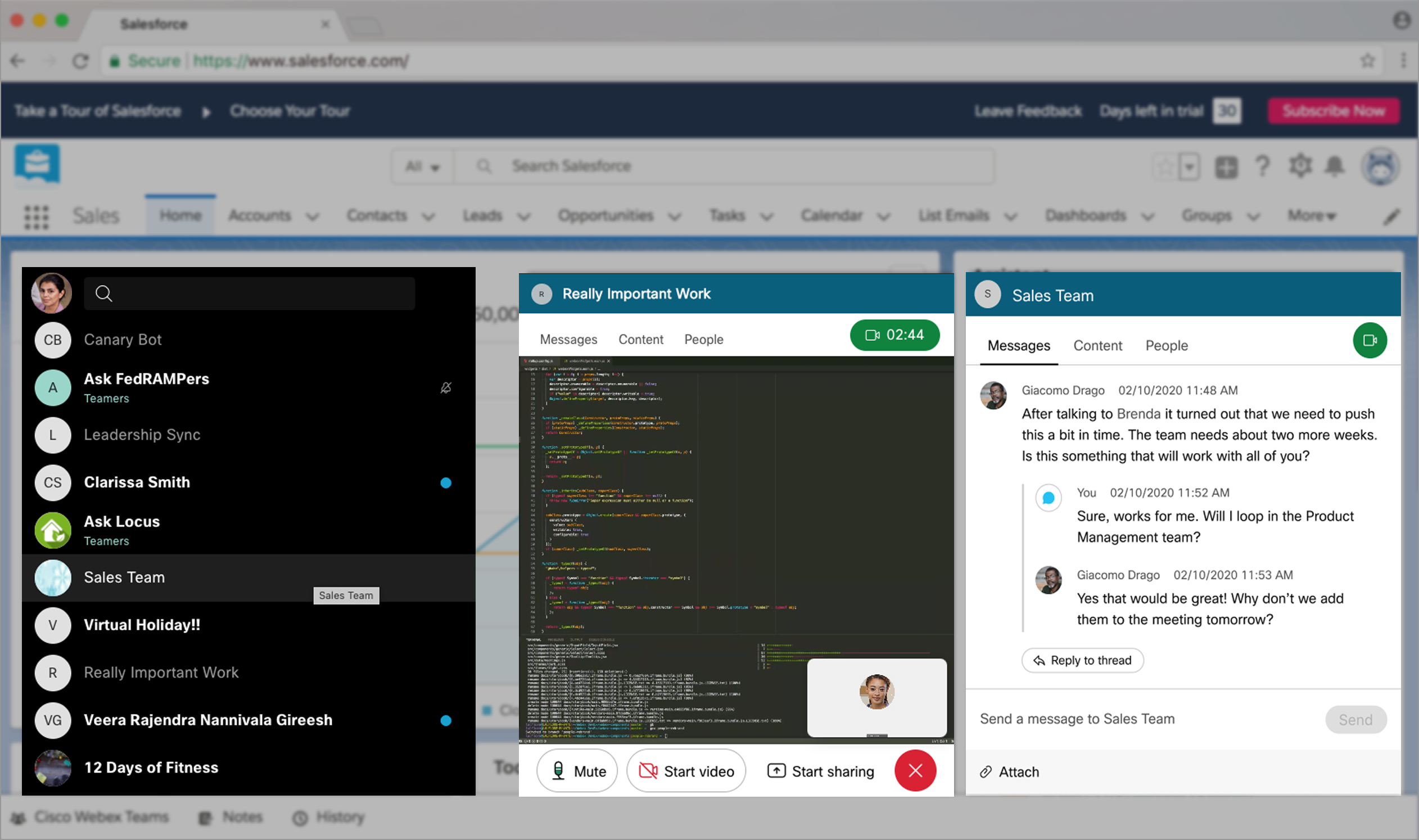
Widgets can be embedded directly in web applications with few lines of code, giving incredible flexibility to many different collaboration workflows without missing out on the Webex features we all love. Widgets come pre-built with the Webex User Interface (UI).
They can be used by either current Webex users or guests by authenticating with an auth token provided from an integration or a guest issuer app.
Today there are three different embeddable experiences available: Space Widget, Recents Widget, and Meetings Widget.
anchorSpace Widget
anchorInclude Webex space functionality in your app. This widget provides messaging, meeting and content sharing features related to spaces. Join 1:1 or group spaces to collaborate via messaging or start and join meetings, all with the familiar Webex UI.
Space Widget Features
-
Messaging:
- 1:1 and group messaging
- Markdown message support
- File sharing. (150MB file size limit)
- Delete/flag messages
- Read receipts
- Persistent chat
- Roster list and @mention
-
Meetings:
- Join 1:1/Group Space meetings using password or hostkey based authentication
- Dial by email address or SIP address. Note: SIP addresses are only supported for Webex meetings SIP URIs and not for Webex Calling or 3rd party SIP URIs
- Share screen or a specific application/browser tab. Note: Safari browsers ONLY support sharing the whole screen
- FedRAMP environment support. See the usage README.
Space Widget Usage
To use this widget, you'll first need to create an integration with the following scopes: spark:all
.
Using our CDN requires the least amount of work to get started. Add the following to your HTML file:
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://code.s4d.io/widget-space/production/main.css">
<!-- Latest compiled and minified JavaScript -->
<script src="https://code.s4d.io/widget-space/production/bundle.js"></script>
You can then instantiate the widget by providing an ACCESS_TOKEN
and SPACE_ID
:
<div id="my-space-widget" />
<script>
// Grab DOM element where widget will be attached
var widgetEl = document.getElementById('my-space-widget');
// Initialize a new Space widget
webex.widget(widgetEl).spaceWidget({
accessToken: 'ACCESS_TOKEN',
destinationType: 'spaceId',
destinationId: 'SPACE_ID'
});
</script>
If you'd like to use a guest token instead of an access token, use the guestToken
parameter with a guest token instead of accessToken
.
For more usage and configuration information, see the Space Widget Usage documentation in GitHub.
Space Widget Requirements
Supported Desktop Browsers: Chrome (latest), Firefox (latest), and Safari (latest).
Please note that Space Widget is not supported on Mobile browsers.
anchorRecents Widget
anchorInclude a user's most recent Webex spaces in your app and respond to real-time events. This widget displays a list of recent Webex spaces and can act as a central event processing hub for events happening within the Webex Platform.
Recents Widget Features
- Recent Webex conversation list (spaces & people)
- Hooks to open a conversation with the Space Widget
- Unread space highlighting
- Event hooks for messages, meetings, and memberships
- Incoming call notifications
- FedRAMP environment support. See the usage README.
Recents Widget Usage
To use this widget, you'll first need to create an integration with the following scopes: spark:all
.
Using our CDN requires the least amount of work to get started. Add the following to your HTML file:
<!-- Production compiled and minified CSS -->
<link rel="stylesheet" href="https://code.s4d.io/widget-recents/production/main.css">
<!-- Production compiled and minified JavaScript -->
<script src="https://code.s4d.io/widget-recents/production/bundle.js"></script>
You can then instantiate the widget by providing an ACCESS_TOKEN
:
<div id="my-recents-widget" />
<script>
// Grab DOM element where widget will be attached
var widgetEl = document.getElementById('my-recents-widget');
// Initialize a new Recents widget
webex.widget(widgetEl).recentsWidget({
accessToken: 'ACCESS_TOKEN'
});
</script>
If you'd like to use a guest token instead of an access token, use the guestToken
parameter with a guest token instead of accessToken
.
For more usage and configuration information, see the Recents Widget Usage documentation in GitHub.
Recents Widget Events
The Recents Widget exposes a few events for hooking into widget functionality. When instantiating a Recents widget, you can provide a callback parameter that will fire whenever an event occurs. You can then filter the actions and perform actions:
<div id="my-recents-widget" />
<script>
// Grab DOM element where widget will be attached
var widgetEl = document.getElementById('my-recents-widget');
// Initialize a new Recents widget
webex.widget(widgetEl).recentsWidget({
accessToken: 'ACCESS_TOKEN',
onEvent: callback
});
function callback(name, detail) {
if (name === 'messages:created') {
// Perform an action if a new message has been created
}
}
</script>
See the Recents Widget Events documentation in GitHub for more information about event handling.
Recents Widget Requirements
Supported Desktop Browsers: Chrome (latest), Firefox (latest), and Safari (latest)
Please note the Recents Widget is not supported on Mobile browsers.
anchorMeetings Widget 🆕
anchorInclude Webex Meetings functionality into your application to join any Webex meeting type, share your screen, manage your media devices and more. The Meetings Widget comes in two themes: Dark and Light and is designed to be responsive, which gives you maximum flexibility on where it can be used!
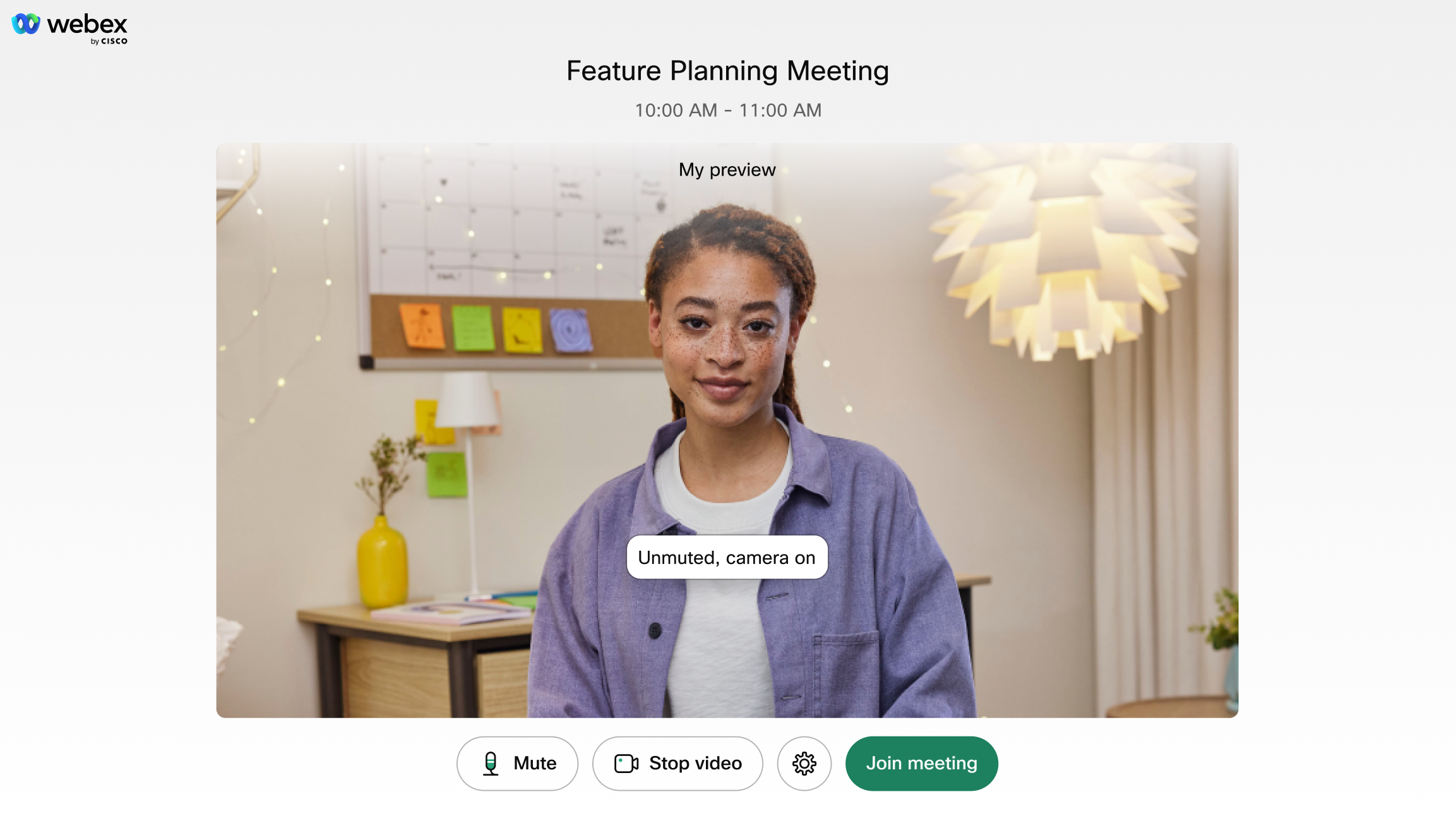
Meetings Widget Features
Meetings
- Join 1:1 or group Space meetings, Personal Meeting Rooms and Scheduled meetings with password or hostkey based authentication.
- Dial by email address, people ID, room ID or SIP address Note: SIP addresses are supported for Webex meetings SIP URIs and Webex cloud-registered devices. Webex Calling or third-party SIP URIs are not supported
- In-meeting participant roster. See participants from your organization and those from outside
- Share your screen or a specific application/browser tab Note: Screen sharing is a desktop-browser-only feature (See compatibility table). MacOS Safari only supports sharing the whole screen
- FedRAMP environment support. See the usage README.
Media Management
- List available audio/video devices
- Mute/Unmute your audio/video before joining and during the meeting
- Switch your audio/video device before joining and during the meeting
Customization
- Choose between the amazing Dark or Light Webex experiences
- Show/hide meeting controls (mute, settings, participants list, screen share, etc.) based on your use case
- Decide which remote video layout (e.g. grid, stack, focus) is best for your application
Accessibility
- Screen-reader ready
- Keyboard navigation
Widget Usage
Meetings Widget is available on NPM with no support of distribution via CDN at the moment.
To get started with the Meetings widget, install the widget package and its dependencies.
npx install-peerdeps @webex/widgets
Import widget package and styles, instantiate a new widget by providing an ACCESS_TOKEN
and a MEETING_DESTINATION
:
import {WebexMeetingsWidget} from '@webex/widgets';
import '@webex/widgets/dist/css/webex-widgets.css';
export default function App() {
return (
<WebexMeetingsWidget
style={{width: "1000px", height: "500px"}} // Substitute with any arbitrary size or use `className`
accessToken="<ACCESS_TOKEN>"
meetingDestination="<MEETING_DESTINATION>"
/>
);
}
See Accounts and Authentication on how to obtain an access token. For production use, you will need to create an integration with the following scopes: spark:all
.
At the moment, guest tokens are not supported directly, but you may use the access token provided after guest token exchange flow.
You can also get started with our Meetings widget sample repository.
Meetings Widget Requirements
- Supported Desktop Browsers: Chrome (latest), Firefox (latest), Edge (latest) and Safari (latest)
- Supported Mobile Browsers: Chrome on Android (latest) and Safari on iOS (latest)
Meetings widget general availability support starts on version 1.23.0 onwards. However, we encourage you to use use the latest version at the time and update regularly, as there will be tons of improvements and bug fixes!
anchorContent Security Policy
anchorWebex Widgets can be used on websites which implement a Content Security Policy (CSP) via the Content-Security-Policy HTTP header. Consult your webserver's documentation for more information about configuring CSP. If you're using CSP, use these individual policy directives and their associated values to enable access to the widgets and to allow connectivity to the Webex Platform:
Policy Directive | Value |
---|---|
script-src | 'self' 'unsafe-inline' https://code.s4d.io; |
style-src | 'self' 'unsafe-inline' https://code.s4d.io; |
media-src | 'self' https://code.s4d.io https://*.clouddrive.com https://*.giphy.com https://*.webexcontent.com data: blob:; |
font-src | 'self' https://code.s4d.io; |
img-src | 'self' https://*.clouddrive.com https://code.s4d.io https://*.webexcontent.com data: blob: https://*.rackcdn.com; |
connect-src | 'self' localhost ws://localhost:8000 wss://*.ciscospark.com wss://*.wbx.com wss://*.wbx2.com https://*.ciscospark.com https://*.clouddrive.com/ https://code.s4d.io https://*.giphy.com https://*.wbx2.com https://*.webex.com https://*.webexcontent.com; |
anchorSupport Policy
anchorFor information on the Webex support policy, see the SDK and API Support Policy.